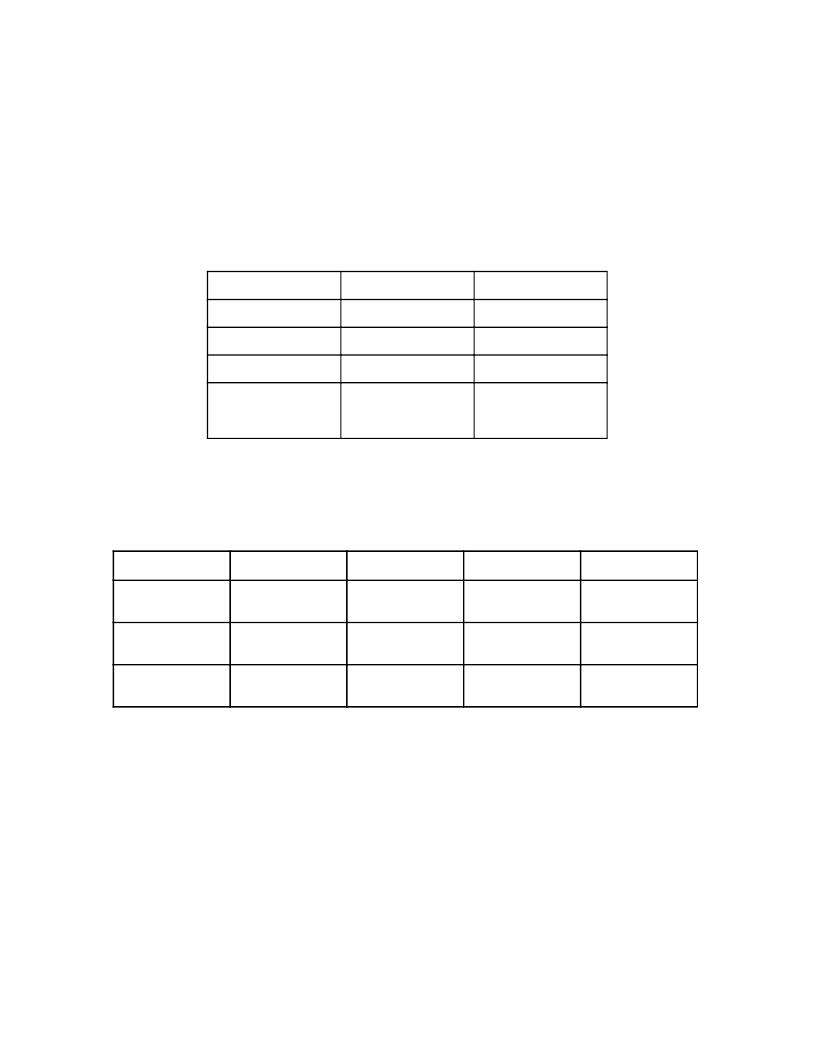
4-7
Programming the DM5806
This section gives you some general information about programming and the DM5806 board, and then walks
you through the major DM5806 programming functions. These descriptions will help you as you use the example
programs included with the board. All of the program descriptions in this section use decimal values unless other-
wise specified.
The DM5806 is programmed by writing to and reading from the correct I/O port locations on the board. These
I/O ports were defined in the previous section. Most high-level languages such as BASIC, Pascal, C, and C++, and
of course assembly language, make it very easy to read/write these ports. The table below shows you how to read
from and write to I/O ports using some popular programming languages.
Language
Modulus
Integer Division
AND
OR
C
%
a = b % c
/
a = b / c
&
a = b & c
|
a = b | c
Pascal
MOD
a := b MOD c
DIV
a := b DIV c
AND
a := b AND c
OR
a := b OR c
BASIC
MOD
a = b MOD c
\
a = b \ c
AND
a = b AND c
OR
a = b OR c
In addition to being able to read/write the I/O ports on the DM5806, you must be able to perform a variety of
operations that you might not normally use in your programming. The table below shows you some of the operators
discussed in this section, with an example of how each is used with Pascal, C, and BASIC. Note that the modulus
operator is used to retrieve the least significant byte (LSB) of a two-byte word, and the integer division operator is
used to retrieve the most significant byte (MSB).
Many compilers have functions that can read/write either 8 or 16 bits from/to an I/O port. For example, Turbo
Pascal uses
Port
for 8-bit port operations and
PortW
for 16 bits, Turbo C uses
inportb
for an 8-bit read of a port
and
inport
for a 16-bit read.
Be sure to use only 8-bit operations with the DM5806!
Language
Read
Write
BASIC
Data=INP(Address)
OUT Address,Data
Turbo C
Data=inportb(Address)
outportb(Address,Data)
Turbo Pascal
Data:=Port[Address]
Port[Address]:=Data
Assembly
mov dx,Address
in al,dx
mov dx,Address
mov al,Data
out dx,al