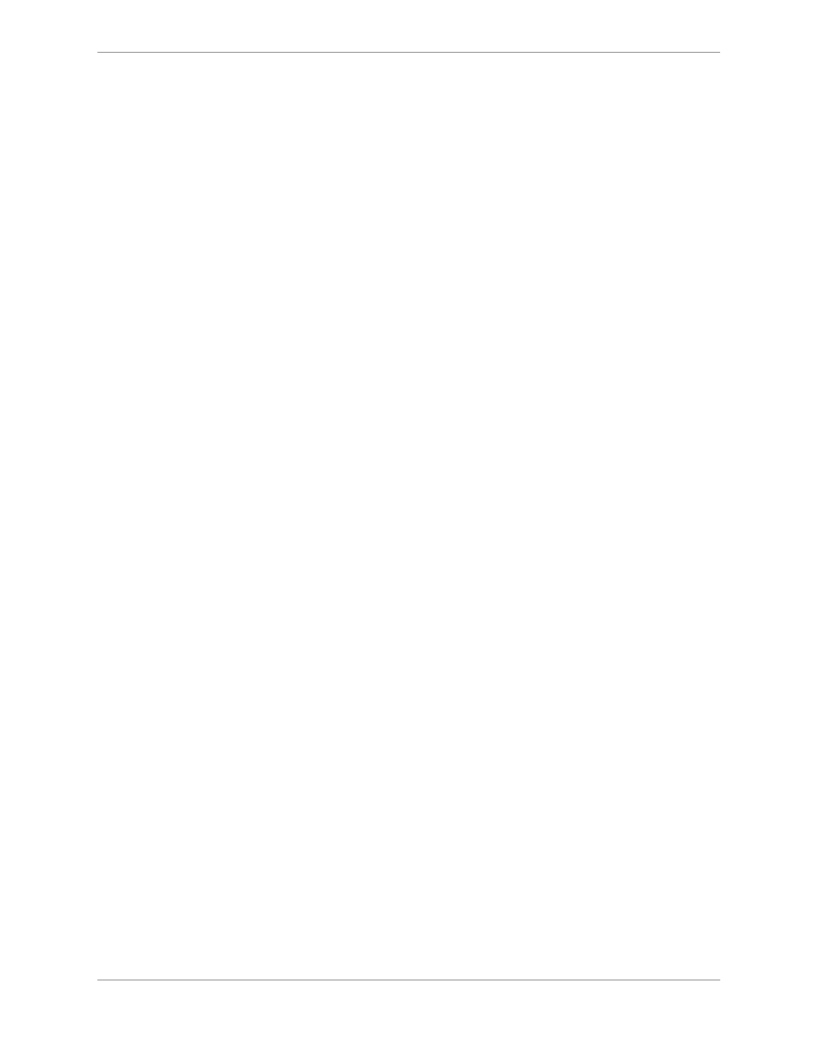
5-44
SC100 C Compiler
Optimization Techniques and Hints
5.4.3.5 Resource limitations
The SC100 architecture provides a total of 16 Dn registers and 16 Rn registers. If the number of active
variables is greater than the number that the registers can accommodate, the compiler maps the extra
variables to memory, resulting in less efficient code.
For best results, you should take account of these physical limitations when writing your source code. For
example, when preparing a set of instructions to execute in one cycle, remember that there is a restriction
on the number of operands that can be used in a single cycle.
5.5 Optimizer Assumptions
The optimizer uses the information passed to it by the compiler, in order to ensure that the optimizations
applied during the various optimization stages do not affect the original accuracy of the program.
At the time that the compiler accumulates this information, it assumes that only two types of variables can
be accessed while inside a function, either indirectly through a pointer or by another function call:
Global variables, meaning all variables within the file scope or application scope
Local variables, whose addresses are retrieved implicitly by the automatic conversion of array
references to pointers, or explicitly by the
&
operator
If your programs conform to the standard ANSI/ISO version of C, this assumption does not affect your
code. If the code that you are compiling is not standard, and it violates this assumption, the optimization
process may adversely affect the behavior of the program.
To avoid unexpected results, and to ensure that your program executes correctly once optimized, follow
the coding guidelines listed below:
Don
’
t make assumptions based on memory layout when using pointers. For example, if
x
points to
the first member of a structure,
x+1
may not necessarily point to the second member of the same
structure. Similarly, if
y
is defined as a pointer to the first declared variable in a list, do not assume
that
y+1
points to the second variable in the list.
When referencing an array, keep the references inside the array bounds.
Ensure that all the required arguments are passed to functions.
When subscribing one array, don
’
t access another array indirectly. For example, if in the construct
x[y-x]
,
x
and
y
are the same type of array, the construct is equivalent to
*(x+(y-x))
, which is
equivalent to
*y
. Thus the construct actually references the array
y
.
When pointing to objects, don
’
t reference outside the bounds of these objects. The optimizer
assumes that all references of the form
*(p+i)
apply within the bounds of the variable(s) to which
p
points.
When the need arises for variables that are accessed by external processes, be sure to declare the
variables as
volatile
. Use this keyword judiciously, as it may have adverse effects on
optimization.