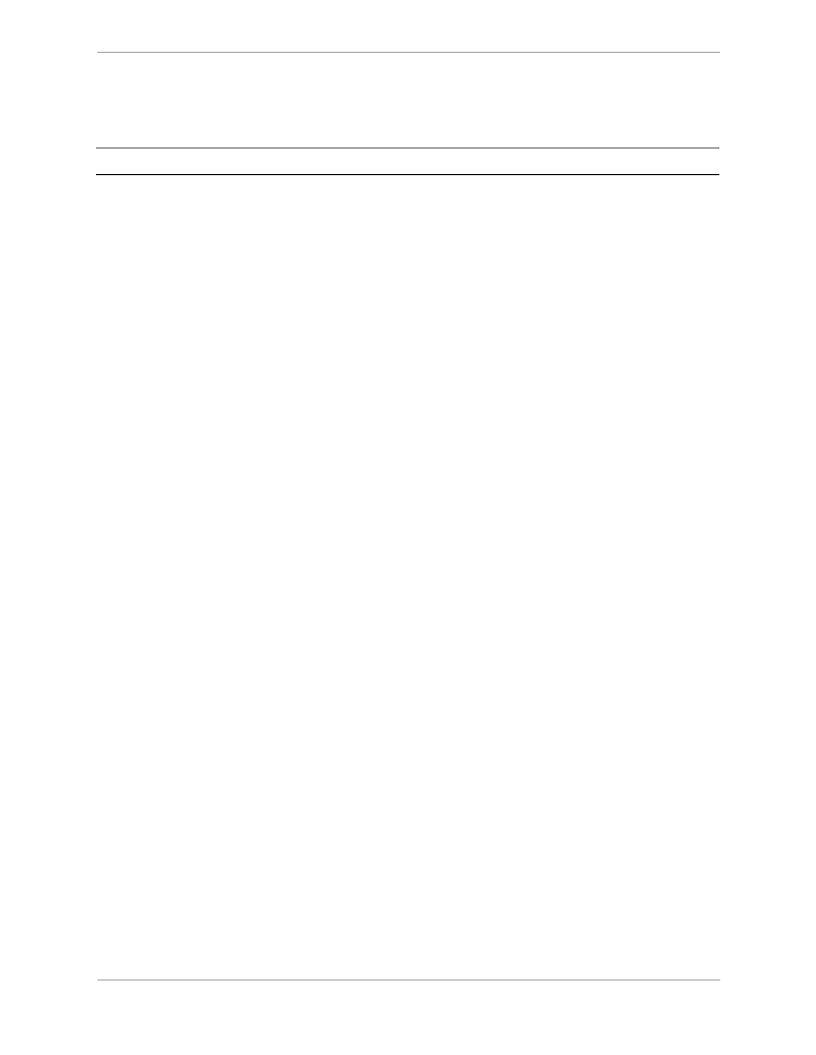
4-2
SC100 C Compiler
Interfacing C and Assembly Code
To inline a single assembly instruction, use the
asm
statement. The syntax is as for a standard function call,
with one argument enclosed in double quotation marks, as shown below in Example 4-1.
Example 4-1. Inlining a single assembly instruction
asm("wait");
4.2 Inlining a Sequence of Assembly Instructions
It is possible to use assembly code that references C objects, by defining a separate function that consists of
a sequence of assembly instructions, and inlining this in your C code. Such a function is implemented
entirely in assembly and may not include C statements, but can accept parameters referenced by the
assembly code.
4.2.1 Guidelines for Inlining Assembly Code Sequences
The following guidelines are similar to those for the inlining of individual assembly instructions, described
in
Section 4.1,
“
Inlining a Single Assembly Instruction,
”
and apply also to the use of inlined sequences of
assembly code:
The compiler passes a sequence of inlined instructions to the assembly output file as a string of text,
and therefore has no knowledge of the contents or side effects of the instructions. It is important that
you ensure that the assembly function does not affect the C and/or assembly environment and does
not produce unpredictable results. For example, do not use inlined assembly instructions to change
the contents of registers, and do not alter the sequence of C code instructions by specifying jumps,
as the compiler has no knowledge of such changes.
Functions based on inlined sequences of assembly code cannot be used by the optimizer, and are
ignored during optimization. Avoid using assembly-based functions if a C alternative is available, in
order to ensure maximum optimization of the code.
The compiler performs no error checking on the sequence of assembly instructions. Assembly code
errors are identified only at the assembly stage of the compilation process.
The guidelines listed below apply specifically to the use of inlined sequences of assembly code:
When passing parameters to an inlined sequence of assembly instructions, registers are not
automatically allocated. You must specify for each parameter the register in which the parameter
enters or exits the function. There is no need to save and restore the registers before and after the
function.
The compiler is unable to deduce whether an inlined function is likely to affect the application, for
example, if it modifies global variables. It is important that you provide the compiler with this
information if there is a possibility that the function may have any side effects.
A function that is initially defined as stand-alone may in certain circumstances be included in another
sequence of instructions. Inlined functions should therefore not use statements such as RTS. If the
function is used in a sequence of instructions, the compiler adds the necessary return statements
automatically.