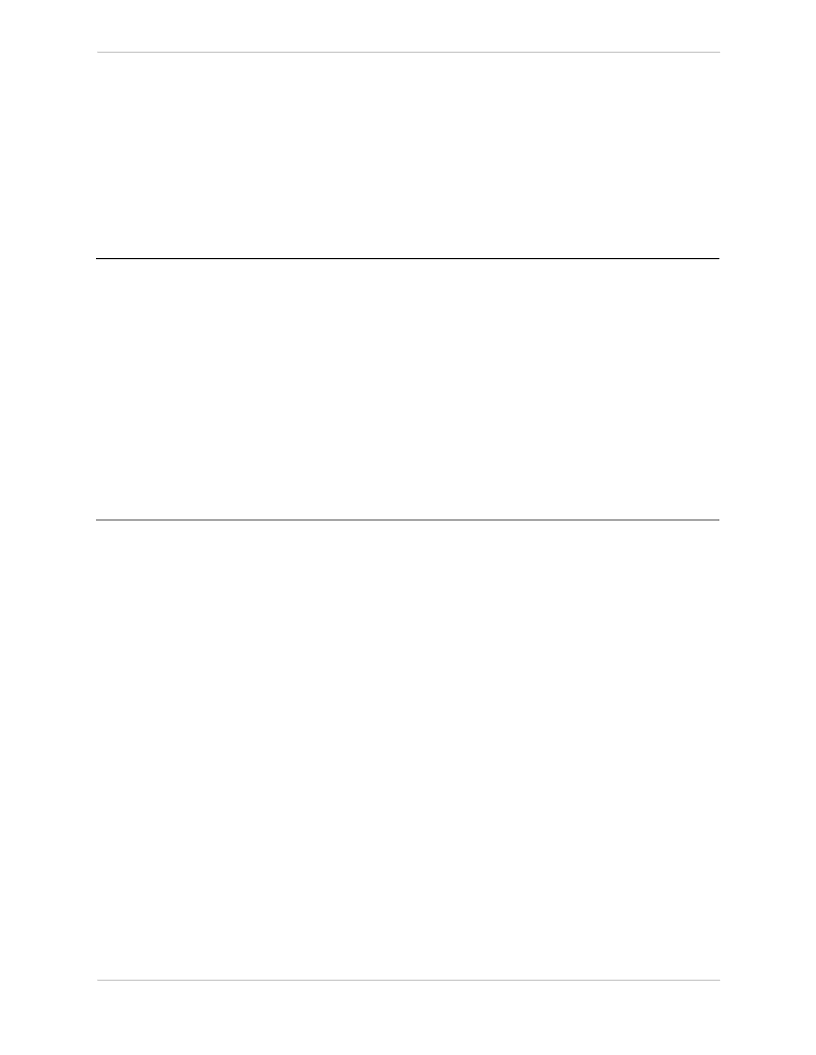
Language Features
SC100 C Compiler
3-59
With
if-then-else
constructs,
#pragma profile
can be used to inform the compiler which branch
executes more frequently, and the frequency ratio between the two branches, meaning the number of times
one branch executes in relation to the other.
In Example 3-34, the two
#pragma profile
statements have the values 5 and 50. These values notify
the compiler that the
else
branch section executes 10 times more frequently than the first (implied
then
)
section. When used in this way, the exact
#pragma profile
values are not significant, since they
indicate the frequency ratio, and not the absolute values. In this example, the values 1 and 10 would
convey the same information.
Example 3-34. #pragma profile with frequency ratio
#include <prototype.h>
int energy (short block[], int N)
{
int i;
long int L_tmp = 0;
if ( N>50)
#pragma profile 5
for (i = 0; i < 50; i++)
L_tmp = L_mac (L_tmp, block[i], block[i]);
else
#pragma profile 50
for (i = 0; i < N; i++)
L_tmp = L_mac (L_tmp, block[i], block[i]);
return round (L_tmp);
}
3.4.5.4.2 Defining a loop count
The compiler tries to evaluate the number of times a loop iterates using the static information available. In
cases where this static information is not supplied to the compiler, if you know the upper and lower limits
of a loop, you can use
#pragma
loop_count
to provide these values. Supplying such information, which
cannot always be discerned automatically by the compiler, enables generation of more efficient code.
Similarly, specifying a divider for the loop count enables the optimizer to unroll loops in the most efficient
way. The loop count can be divided by either 2 or 4, corresponding to the number of execution units. You
can also instruct the compiler whether to use the remainder, if there is one following division of the loop
count, to execute the loop an additional number of times.
The syntax of
#pragma
loop_count
is:
#pragma loop_count (lower_bound, upper_bound, [{2/4}, [remainder]]
)
Define a value for lower_bound for the minimum number of times the loop will iterate, and a value for
upper_bound
for the maximum number of times.
The divider parameter is optional. Only the values 2 or 4 may be specified as the divider.
To specify that a remainder should be used for the loop count, specify a value for
remainder
. The
remainder
argument is only valid if a value has been specified for the divider.