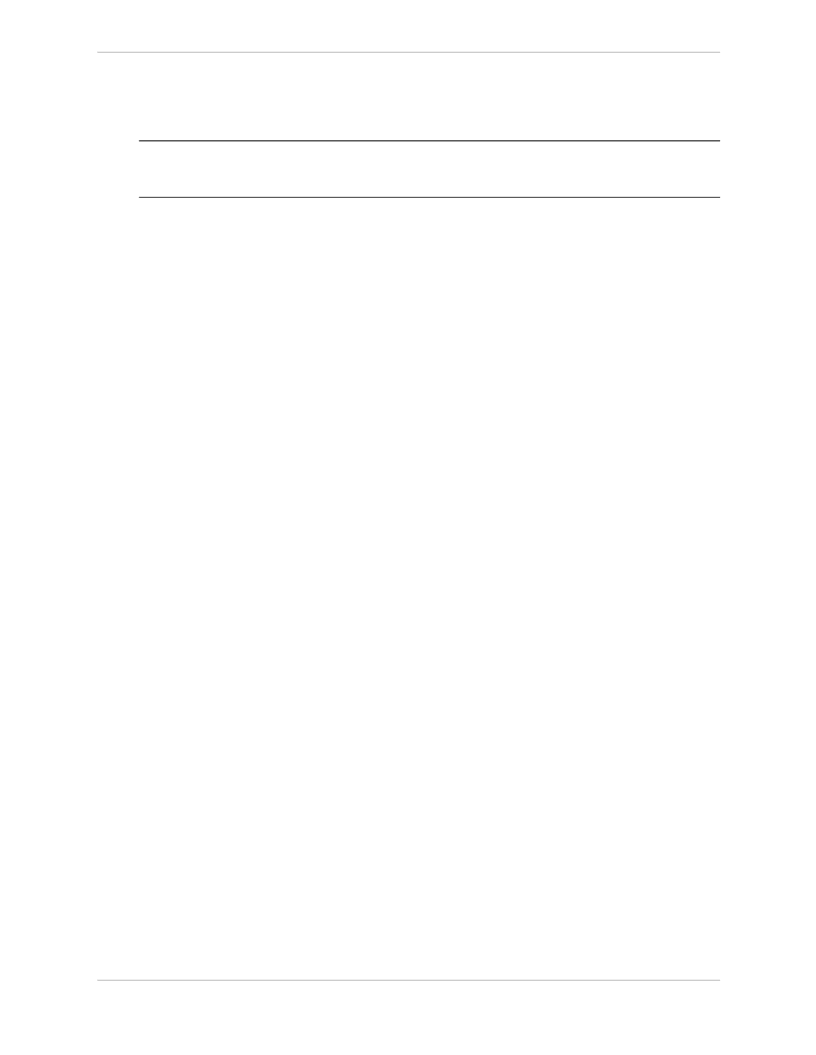
Language Features
SC100 C Compiler
3-29
An
asm
function must be declared with no storage class, with a prototyped parameter list, and with
no omitted parameters, as shown in Example 3-11:
Example 3-11. Declaring an asm function
asm void f(int,int) {
...
}
As an
asm
function must be output with a prototyped parameter list, these functions are valid for
ANSI C modes only.
3.4.1.1.4 Types
The following type extensions are accepted:
Bit-fields may have base types that are
enums
or integer types, as well as the types
int
and
unsigned
int
. The use of any signed integer type is equivalent to using type
int
, and the use of
any unsigned integer type is equivalent to using type
unsigned
int.
The last member of a
struct
may have an incomplete array type. It may not be the only member
of the
struct
(otherwise, the
struct
would have zero size).
A file-scope array may have an incomplete
struct
,
union
, or
enum
type as its element type. The
type must be completed before the array is subscripted (if it is subscripted), and by the end of the
compilation if the array is not
extern
.
The
enum
tags may be incomplete. The tag name can be defined and resolved later (by specifying
the brace-enclosed list).
Object pointer types and function parameter arrays that decay to pointers may use
restrict
as a
type qualifier. Its presence is recorded in the compiler so that optimizations can be performed that
would otherwise be prevented because of possible aliasing.
The type
long float
is accepted as a synonym for
double
.
Assignment of pointer types is allowed in cases where the destination type has added type qualifiers
that are not at the top level (for example,
int **
to
const int **
).
3.4.1.1.5 Expressions and statements
The following extensions are accepted for expressions and statements:
Assignment and pointer differences are allowed between pointers to types that are interchangeable,
but not identical, for example,
unsigned char *
and
char *
. This includes pointers to
same-sized integral types (e.g., typically,
int *
and
long *
). A warning is issued, except in
pcc
mode. A string constant may be assigned to a pointer to any kind of character, without a warning.
In operations on pointers, a pointer to
void
is always implicitly converted to another type if
necessary, and a null pointer constant is always implicitly converted to a null pointer of the right type
if necessary. In ANSI C, some operators allow such conversions, while others do not, generally
where such a conversion would not be logical.
In an initializer, a pointer constant value may be cast to an integral type if the integral type is big
enough to contain it.
In an integral constant expression, an integer constant may be cast to a pointer type and then back to
an integral type.
In character and string escapes, if the character following the
\
has no special meaning, the value
of the escape is the character itself. Thus
“
\s
”
==
“
s
”
. Awarning is issued.