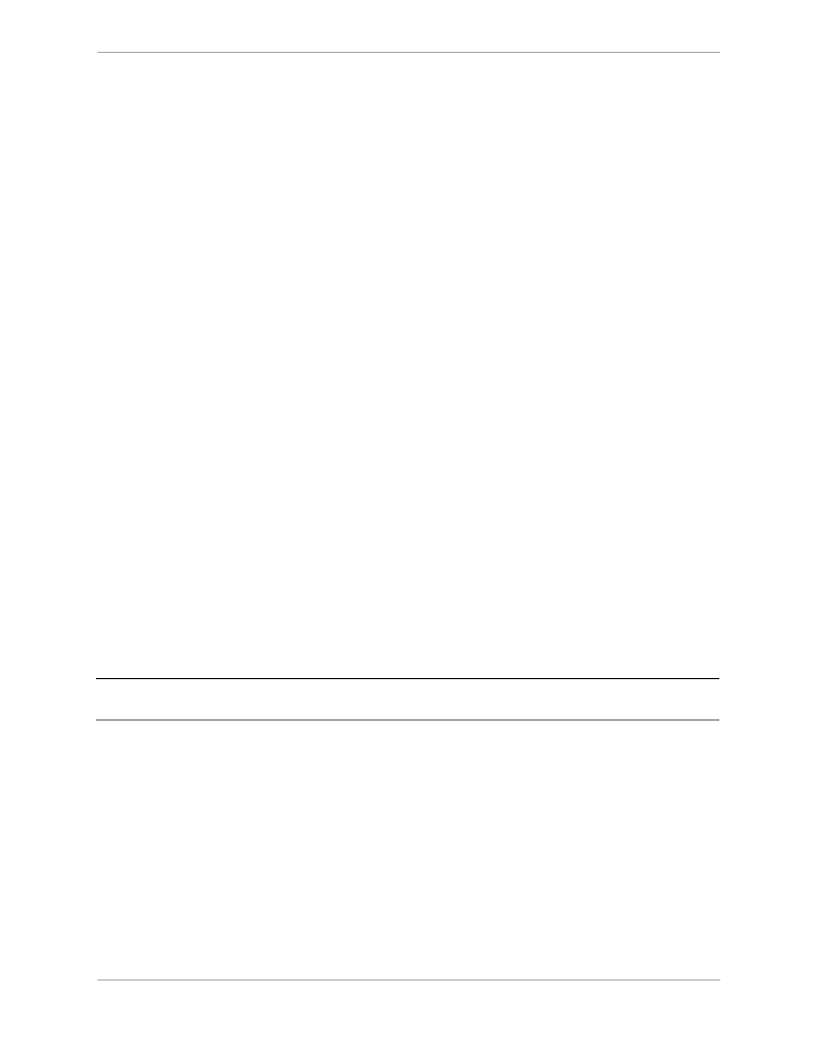
6-8
SC100 C Compiler
Runtime Environment
The compiler makes no assumptions about which stack pointer to use, and uses the pointer for the current
processor mode to point to the address at the top of the stack.
When the system is initialized, the stack pointer for the current mode is set by default to the address of the
location directly after the code area, as defined in
StackStart
in the linker command file. The actual
address of the stack is determined at link time.
The stack pointer for the current processor mode is automatically incremented by the C environment at the
entry to a function. This ensures that sufficient space is reserved for the execution of the function. At the
function exit, the stack pointer is decremented, and the stack is restored to its previous size prior to
function entry. If your application includes assembly language routines and C code, you must ensure at the
end of each assembly routine that the current stack pointer is restored to its pre-routine entry state.
Note:
If you change the default memory configuration, remember to allow sufficient space for the stack
to grow. If a stack overflow occurs at runtime, this will cause your program to fail. The compiler
does not check for stack overflow during compilation or at runtime.
6.3.1.2 Dynamic memory allocation (heap)
The runtime libraries supported by the compiler include a number of functions which enable you to
allocate memory dynamically for variables. See Chapter 7 for details of the runtime libraries supported.
Since C does not support the dynamic allocation of memory, the compiler assigns an area of memory as a
heap for this purpose.
The compiler allocates memory from a global pool for the stack and the heap together. The lower address
of the area assigned to the stack and heap is defined in
StackStart
, in the linker command file. The heap
starts at the top of memory, and is allocated in a downward direction toward the stack.
Objects that are dynamically allocated are addressed only with pointers, and not directly. The amount of
space that can be allocated to the heap is limited by the amount of available memory in your system.
To make more efficient use of the space allocated to data, you can use the heap to allocate large arrays,
instead of defining them as static or global.
For example, a definition such as
struct large array1[80];
can be defined using a pointer and the
malloc
function, as illustrated in Example 6-6.
Example 6-6. Allocating large arrays from the heap
struct large *array1;
array1 = (struct large *)malloc(80*sizeof(struct large));
6.3.2 Static Data Allocation
When you compile your application in non-global mode, the allocations for each file are assigned to
different sections of data memory. At link time these are dispatched to different addresses.
When compiling in global mode, the compiler uses the same data section for all allocations. If you want to
override this and to instruct the compiler to use non-contiguous data blocks, you can edit the machine
configuration file to define the exact memory map of the system that you want to use. For more details, see
Section 6.3.4,
“
Machine Configuration File.
”